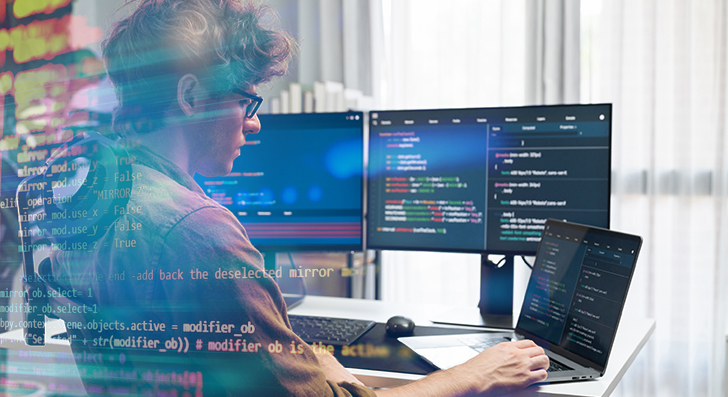
Scalability indicates your application can deal with growth—extra people, a lot more information, and much more traffic—without the need of breaking. Like a developer, developing with scalability in your mind saves time and stress later on. Right here’s a transparent and functional guidebook that can assist you begin by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later on—it ought to be element within your approach from the beginning. Lots of programs are unsuccessful after they grow rapidly because the initial design can’t tackle the extra load. For a developer, you have to Assume early about how your technique will behave stressed.
Commence by coming up with your architecture being flexible. Keep away from monolithic codebases where every little thing is tightly related. Rather, use modular layout or microservices. These styles break your app into smaller, independent areas. Each individual module or support can scale By itself without the need of affecting The entire process.
Also, think about your databases from working day a single. Will it need to have to take care of a million customers or maybe 100? Pick the correct variety—relational or NoSQL—based upon how your details will grow. Program for sharding, indexing, and backups early, Even though you don’t need to have them nonetheless.
Another essential issue is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest disorders. Think about what would happen if your user base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design styles that aid scaling, like information queues or party-pushed devices. These enable your application tackle extra requests without the need of having overloaded.
After you Make with scalability in your mind, you're not just preparing for success—you are decreasing future problems. A perfectly-prepared program is easier to take care of, adapt, and mature. It’s superior to get ready early than to rebuild afterwards.
Use the ideal Databases
Deciding on the suitable databases is often a essential Portion of developing scalable applications. Not all databases are built a similar, and utilizing the Improper one can gradual you down as well as result in failures as your application grows.
Start out by comprehension your knowledge. Is it remarkably structured, like rows within a table? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They're strong with interactions, transactions, and regularity. They also guidance scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted traffic and information.
If the information is a lot more adaptable—like user action logs, product catalogs, or paperwork—consider a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and can scale horizontally extra effortlessly.
Also, look at your study and publish styles. Have you been executing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a heavy compose load? Check into databases that may manage significant write throughput, and even celebration-centered information storage techniques like Apache Kafka (for non permanent information streams).
It’s also wise to Assume in advance. You might not need Superior scaling characteristics now, but picking a databases that supports them means you won’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information dependant upon your entry designs. And constantly watch databases performance as you grow.
In short, the proper database depends upon your app’s structure, velocity requires, And exactly how you hope it to develop. Consider time to pick sensibly—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, each individual little delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the beginning.
Commence by creating clear, easy code. Steer clear of repeating logic and take away nearly anything needless. Don’t choose the most elaborate Option if a simple one is effective. Maintain your features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too extensive to run or uses too much memory.
Upcoming, take a look at your databases queries. These usually gradual items down more than the code by itself. Make sure Every single query only asks for the information you truly will need. Steer clear of Pick out *, which fetches every thing, and in its place choose unique fields. Use indexes to speed up lookups. And keep away from doing a lot of joins, especially throughout large tables.
Should you discover the exact same data getting asked for again and again, use caching. Retailer the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced operations.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app much more productive.
Make sure to exam with large datasets. Code and queries that function great with a hundred records may crash whenever they have to manage one million.
Briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra users and more visitors. If every little thing goes by way of one particular server, it will eventually immediately turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a person server accomplishing the many operate, the load balancer routes consumers to various servers according to availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based solutions from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused speedily. When customers ask for precisely the same details again—like an item webpage or perhaps a profile—you don’t really need to fetch it with the databases each time. You could serve it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) merchants information in memory for rapid entry.
two. Customer-side caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t change typically. And usually be certain your cache is up to date when info does transform.
In a nutshell, load balancing and caching are simple but strong applications. With each other, they assist your application deal with additional users, remain rapid, and recover from difficulties. If you intend to expand, you would like both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application grow effortlessly. That’s the place cloud platforms and containers can be found in. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When targeted visitors improves, you'll be able to incorporate far more methods with just a couple clicks or automatically using auto-scaling. When traffic drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. here You are able to concentrate on developing your application in lieu of running infrastructure.
Containers are A further critical Resource. A container deals your application and almost everything it should run—code, libraries, settings—into one device. This causes it to be straightforward to move your application involving environments, from the laptop to the cloud, without the need of surprises. Docker is the preferred Device for this.
When your application uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it quickly.
Containers also help it become simple to different areas of your app into companies. You are able to update or scale pieces independently, that's great for functionality and dependability.
In short, working with cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature with no limits, commence applying these resources early. They help save time, reduce hazard, and enable you to keep focused on creating, not correcting.
Monitor Almost everything
For those who don’t keep track of your application, you received’t know when things go Incorrect. Checking can help the thing is how your app is executing, place challenges early, and make better choices as your app grows. It’s a vital A part of constructing scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—observe your app too. Keep an eye on how long it will take for customers to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This assists you repair issues fast, frequently prior to users even see.
Checking is additionally helpful whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it results in authentic hurt.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties right up until it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring can help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for massive businesses. Even smaller apps will need a strong Basis. By developing diligently, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Consider big, and Construct clever.